I downloaded an audio file to listen on my cell phone. But the information with the mp3 file was disturbed. Everywhere, there was a dummy website that appeared. Now, If I open it in my cell phone, instead of title of the song, the same website name appeared. It is quite possible that filename contains the real name of song while title and other details have dummy details.
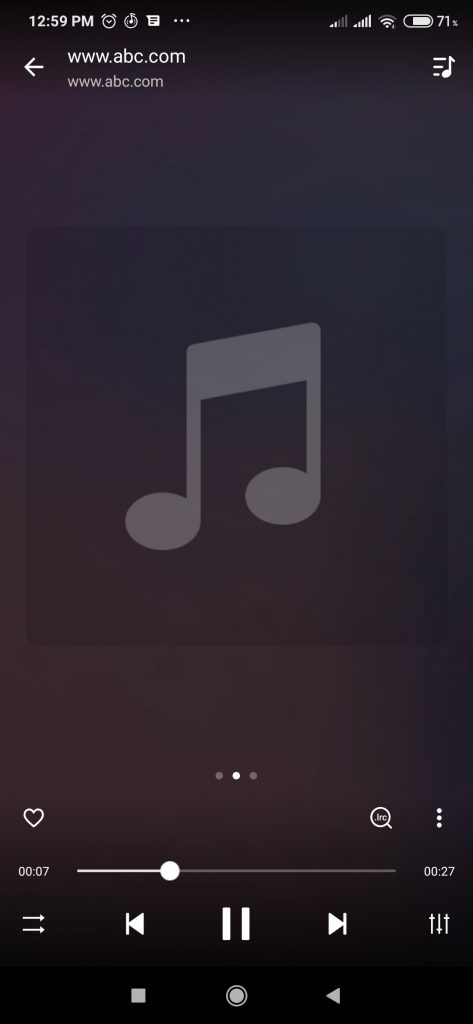
A quick search tells that there are some software available to change the audio tags but… who needs a software when you can write a code in python!
Python is blessed with a library called eyeD3. It is a simple and easy to use library that helps you change titles of your mp3 files easily.
For this tutorial, I am assuming to replace the file name with the title of the song and while other things are not required, we are still going to change it to something nice.
So Lets get started:
Install eyeD3 by executing following command:
pip install eyed3
or if you are using pip3, then use following command:
pip3 install eyed3
then we need to import the library along with basic os library to get files from a folder
import eyed3
import os
Next, we will first read files from the folder where you have put them.
directory = os.listdir()
audios = [x for x in directory if x[-4:]=='.mp3']
In first line above, we listed all the available files in our current directory. If your current directory (where your .py file is) is not in same folder as others, you may pass the exact link to folder in listdir. Note that you will user same paths while reading the file as well. For this tutorial, we have kept the .py file in same folder as the mp3 file itself.
The second line is selecting the mp3 files from the all items present in the director. x[-4:0] reads last 4 characters of the text file.
Now we loop through the files and print the details to see what is printed.
eyed3 needs to load file. I have loaded the file in the audiofile variable.
Now using audiofile.tag class, we just read the audio parameters and print them one by one.
for file in audios:
audiofile = eyed3.load(file) #Load the file
print("ArtistName:",audiofile.tag.artist)
print("AlbumName: ",audiofile.tag.album)
print("AlbumArtistName: ",audiofile.tag.album_artist)
print("TitleName: ",audiofile.tag.title)
print("TrackNumber: ",audiofile.tag.track_num)
print("Genre Name: ",audiofile.tag.genre)
that gives following output for each audio. We are taking one mp3 file so output is repeated once:
ArtistName: www.abc.com AlbumName: www.abc.com AlbumArtistName: www.abc.com TitleName: www.abc.com TrackNumber: (1234567890, None) Genre Name: www.abc.com
As you can see everything is changed to www.abc.com. Now, very easily, we can change it back to what we want to see. we can simply use the tag properties and assign them new values.
for file in audios:
audiofile = eyed3.load(file) #Load the file
audiofile.tag.title = file.replace(".mp3",'')
audiofile.tag.save()
Let me explain what i am doing there. First I loaded the file using eyed3.load. Then I set its title as its filename. Note that file has the extension as well that we dont want in the title. So I am replacing .mp3 with a null string. Dont forget to save the changes done at the end.
Thats it! You are all done. Now its time to change the mp3 file in cell phone and test!
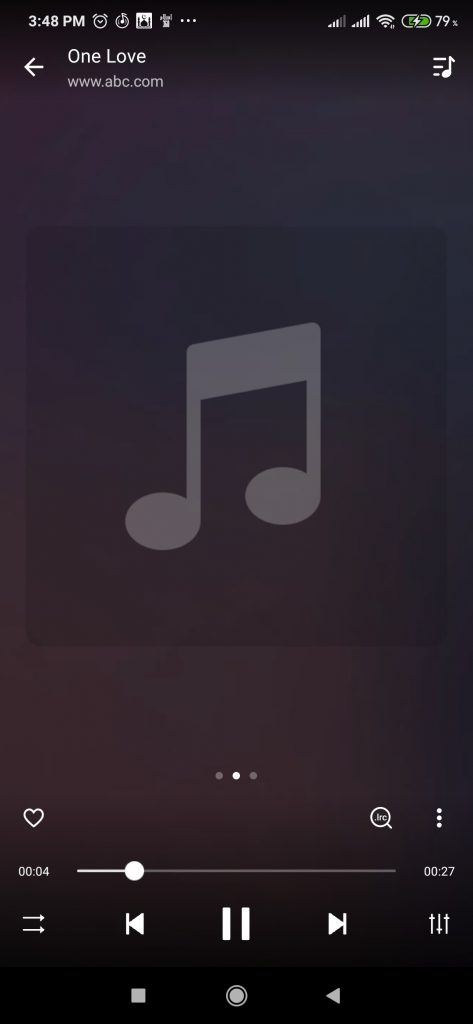
As a challenge, go ahead and change the other features and play around with it. Don’t forget to save at the end! 🙂 Cheers!